前言:项目启动时,需要把数据刷入redis
,在需要的地方使用
maven
引入redis
1 2 3 4 5
| <!--集成redis--> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-redis</artifactId> </dependency>
|
yml
添加redis相关配置
1 2 3 4 5 6 7 8 9 10 11
| spring: redis: database: 2 password: 密码 host: redisIp port: reids端口 jedis: pool: max-active: 10 max-idle: 8 max-wait: 1000
|
数据刷入redis
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; import com.clesun.dao.BaseCoordinateMapper; import com.clesun.entity.BaseCoordinate; import lombok.extern.slf4j.Slf4j; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.boot.CommandLineRunner; import org.springframework.data.redis.core.RedisTemplate; import org.springframework.stereotype.Component;
import java.util.List;
@Slf4j @Component @Slf4j @Component public class DataLoader implements CommandLineRunner {
@Autowired private BaseCoordinateMapper baseCoordinateMapper;
@Autowired private RedisTemplate<String, String> redisTemplate;
@Override public void run(String... args) { log.info("正在执行坐标库刷入redis操作,请勿立即执行导入操作...."); deleteOldCoordinates(); saveCoordinatesToRedis(); log.info("刷入redis操作执行完毕,祝你生活愉快!"); }
private void deleteOldCoordinates() { for (int type = 0; type <= 5; type++) { String key = "coordinateType_" + type; redisTemplate.delete(key); } }
private void saveCoordinatesToRedis() { List<BaseCoordinate> baseCoordinateList = baseCoordinateMapper.selectList(new LambdaQueryWrapper<>()); for (BaseCoordinate coordinate : baseCoordinateList) { String key = "coordinateType_" + coordinate.getType(); redisTemplate.opsForHash().put(key, coordinate.getAddress(), coordinate.getLongitude() + "," + coordinate.getLatitude()); } } }
|
数据结构
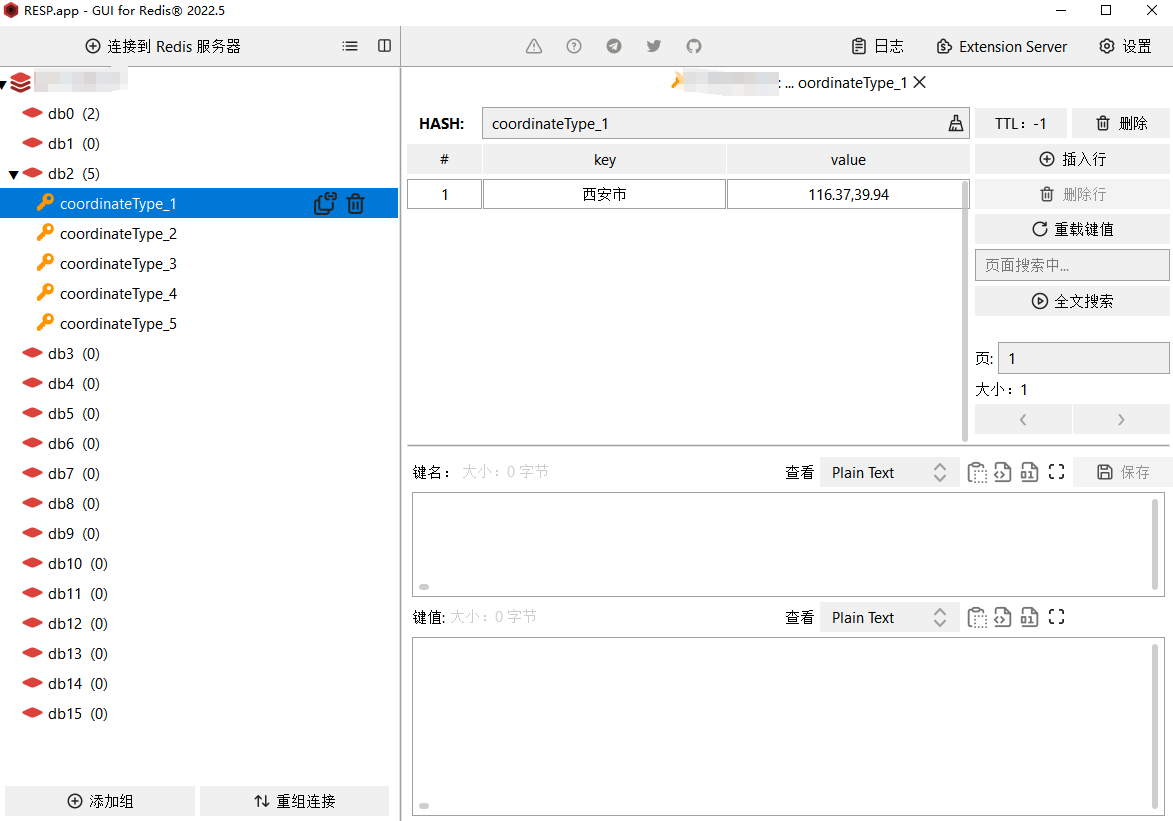
从redis中取数据
从reids中取数据的操作,循环查询redis中的符合要求的key,找到对应的数据
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| public Optional<String> getCoordinate(String address, String... coordinateTypes) { for (String coordinateType : coordinateTypes) { String key = "coordinateType_" + coordinateType; String coordinateString = (String) redisTemplate.opsForHash().get(key, address); if (coordinateString != null) { return Optional.of(coordinateString); } } return Optional.empty(); }
|
将取出来的值,按,分隔开存储进对象中
1 2 3 4 5 6 7
| Optional<String> coordinate = getCoordinate(data.get(5), "5"); if (coordinate.isPresent()) { String coordinateString = coordinate.get(); String[] parts = coordinateString.split(","); entity.setLongitude(parts[0]); entity.setLatitude(parts[1]); }
|